紀錄 PostAsJsonAsync, ReadFromJsonAsync 與 record 型別
參考型別 record
public record AuthResult(
[property: JsonPropertyName("access_token")]
string AccessToken,
[property: JsonPropertyName("expires_in")]
int ExpiresIn,
[property: JsonPropertyName("token_type")]
string TokenType
);
PostAsJsonAsync
& ReadFromJsonAsync
private readonly HttpClient _httpClient;
public AuthService(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task<AuthResult> GetAccessTokenAsync()
{
var response = await _httpClient.PostAsJsonAsync("oauth/token", new
{
grant_type = "client_credentials",
client_id = "client_id",
client_secret = "client_secret"
}
);
response.EnsureSuccessStatusCode();
var result = await response.Content.ReadFromJsonAsync<AuthResult>();
return result;
}
DI
private void ConfigureHttpClient(ServiceConfigurationContext context, IConfiguration configuration)
{
context.Services.AddHttpClient<IAuthService, AuthService>(opt =>
{
var baseAddress = configuration.GetSection(nameof(AuthService))[nameof(opt.BaseAddress)];
if (Uri.TryCreate(baseAddress, UriKind.Absolute, out var uri))
{
opt.BaseAddress = uri;
}
});
}
Test
[Fact]
public async Task Should_Get_AccessToken_From_AuthService()
{
var authService = GetRequiredService<AuthService>();
//Act
var result = await authService.GetAccessTokenAsync();
//Assert
result.ShouldNotBeNull();
result.AccessToken.ShouldNotBeNull();
}
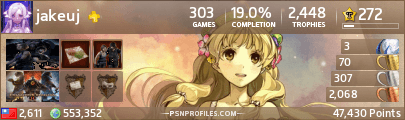